ProductEventListView
This component allows you to integrate the product list at the event level.
How it looks
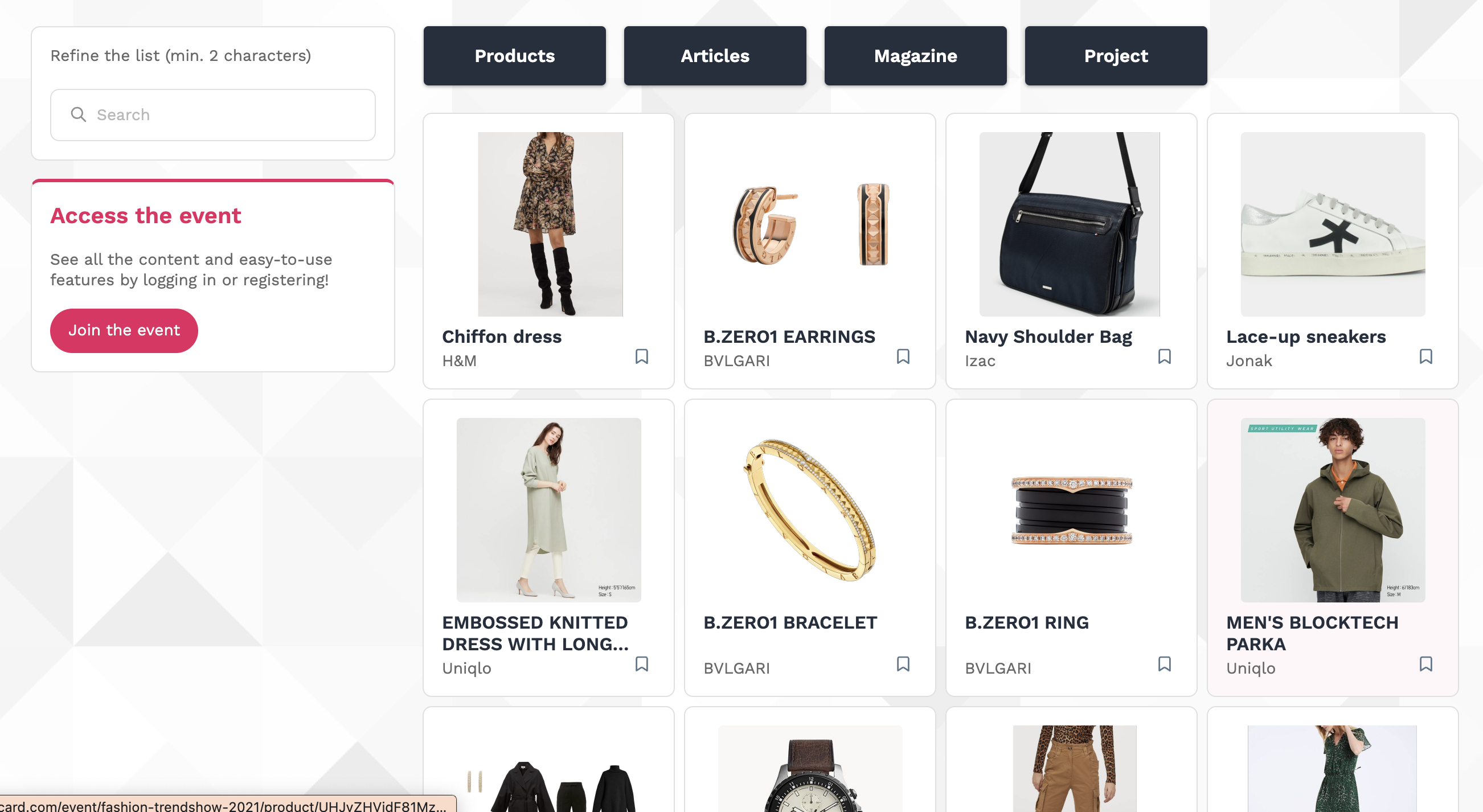
Code sample
import { ProductEventListView } from "@swapcard/react-sdk/lib/product/event-list-view";
export function YourProductListPage() {
return <ProductEventListView viewId="<YOUR_PRODUCT_EVENT_VIEW_ID_HERE>" />;
}
Available props
-
viewId
string (required)
ID of the view you want to render
-
renderProductCard
(node: ReactNode, product: Product) => ReactNode
Render function to wrap each product cells into your own links.
renderProductCard={(node, product) => <a href={`/path/to/product/${product.id}`}>{node}</a>}
-
categoryId
string
ID of the product category
-
renderCategoryLink
(node: ReactNode, category: Category) => ReactNode
Render function to wrap each top navigation category cells into your own links.
renderCategoryLink={(node, category) => <a href={`/products?categoryId=${category.id}`}>{node}</a>}
-
renderViewLink
(node: ReactNode, view: View) => ReactNode
Render function to create the link of your view
renderViewLink={(node, view) => <a href={`/products`}>{node}</a>}
-
search
string
Used to filter result based on user search (to combine with onSearch)
-
onSearch
(search: string) => void
Callback when user searches through the input (to combine with search)
-
selectedFilters
Filter[]
Used to filter result based on user filters (to combine with onFilter)
-
onFilter
(filters: Filter[]) => void
Callback when user filters through checkboxes (to combine with selectedFilters)
-
stickyOffset
number
Search and filter containers will be sticky while the user scrolls (this represents the number in pixel from the top of the body)
-
paginationMode
INFINITE_SCROLL | BUTTON
You can select either if you want pagination to be trigger automatically when the user scroll, or to display a button to force the user to interact to load more elements.